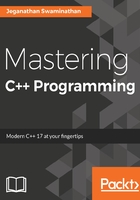
上QQ阅读APP看书,第一时间看更新
Commonly used APIs in an array
The following table shows some commonly used array APIs:

The array container supports random access; hence, given an index, the array container can fetch a value with a runtime complexity of O(1) or constant time.
The array container elements can be accessed in a reverse fashion using the reverse iterator:
#include <iostream>
#include <array>
using namespace std;
int main () {
array<int, 6> a;
int size = a.size();
for (int index=0; index < size; ++index)
a[index] = (index+1) * 100;
cout << "\nPrint values in original order ..." << endl;
auto pos = a.begin();
while ( pos != a.end() )
cout << *pos++ << "\t";
cout << endl;
cout << "\nPrint values in reverse order ..." << endl;
auto rpos = a.rbegin();
while ( rpos != a.rend() )
cout << *rpos++ << "\t";
cout << endl;
return 0;
}
We will use the following command to get the output:
./a.out
The output is as follows:
Print values in original order ...
100 200 300 400 500 600
Print values in reverse order ...
600 500 400 300 200 100