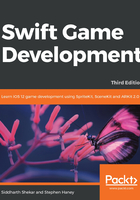
Assigning a physics body to the player
We will use physics forces to move our player around the screen. To apply these forces, we must first add a physics body to the player sprite.
Creating a physics body shape from a texture
When gameplay allows, you should use circles to define your physics bodies—circles are the most efficient shape for the physics simulation and result in the highest frame rate. However, the accuracy of Pierre's shape is very important to our gameplay, and a circle is not a great fit for his shape. Instead, we will assign a special type of physics body, based on his texture.
Apple introduced the ability to define the shape of a physics body with opaque texture pixels in Xcode 6. This is a convenient addition as it allows us to create extremely accurate shapes for our sprites. There is a performance penalty, however, it is computationally expensive to use these texture-driven physics bodies. You will want to use them sparingly, only on your most important sprites.
To create Pierre's physics body, add this code to Player.swift
, at the bottom of the init
function:
// Create a physics body based on one frame of Pierre's animation. // We will use the third frame, when his wings are tucked in let bodyTexture = textureAtlas.textureNamed("pierre-flying-3") self.physicsBody = SKPhysicsBody( texture: bodyTexture, size: self.size) // Pierre will lose momentum quickly with a high linearDamping: self.physicsBody?.linearDamping = 0.9 // Adult penguins weigh around 30kg: self.physicsBody?.mass = 30 // Prevent Pierre from rotating: self.physicsBody?.allowsRotation = false
Run the project and the ground will appear to rise up to Pierre. Since we have given him a physics body, he is now subject to gravity. Pierre is actually dropping down the grid and the camera is adjusting to keep him centered. This is fine for now; we will give him the tools to fly into the sky later. Next, let's learn how to move a character, based on the tilt of the physical device.