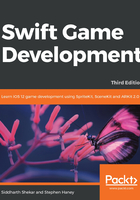
Working with textures
You may want to take a screenshot of your blue box for your own enjoyment later. I absolutely love reminiscing over old screenshots of my finished games when they were nothing more than simple colored blocks sliding around the screen. Now, it is time to move past that stage and attach some fun artwork to our sprite.
Downloading the free assets
I am providing a downloadable pack for all of the art assets I use in this book. I recommend you use these assets so that you will have everything you need for our demo game. Alternatively, you are certainly free to create your own art for your game if you prefer.
More exceptional art assets
If you like the art assets, you can download over 20,000 game assets in the same style for a small donation at http://kenney.itch.io/kenney-donation. I do not have an affiliation with Kenney; I just find it admirable that he has released so much public domain artwork for indie game developers.
These assets are public domain, which means you can copy, modify, and distribute the art assets, even for commercial purposes, all without asking permission. You can read the full license at https://creativecommons.org/publicdomain/zero/1.0/.
Drawing your first textured sprite
Let's use some of the graphics you just downloaded. We will start by creating a bee sprite. We will add the bee texture to our project, load the image onto a SKSpriteNode
class, and then size the node for optimum sharpness on Retina screens.
Adding the bee image to your project
We need to add the image files to our Xcode project before we can use them in the game. Once we add the images, we can reference them by name in our code; SpriteKit is smart enough to find and implement the graphics. Follow these steps to add the bee image to the project:
- Right-click on your project in the project navigator and click Add Files to "Pierre Penguin Escapes the Antarctic" (or the name of your game). Refer to this screenshot to find the correct menu item:
- Browse to the asset pack you downloaded and locate the
bee@3x.png
image inside theEnemies
folder. - Check Copy items if needed, then click Add.
You should now see bee@3x.png
in your project navigator.
Loading images with SKSpriteNode
It is quite easy to draw images to the screen with SKSpriteNode
. Start by clearing out all of the code we wrote for the blue square inside the didMove
function in GameScene.swift
. Replace didMove
with this code:
Override func didMove(to view: SKView) { // Position from the lower left corner self.anchorPoint = .zero // set the scene's background to a nice sky blue // Note: UIColor uses a scale from 0 to 1 for its colors self.backgroundColor = UIColor(red: 0.4, green: 0.6, blue: 0.95, alpha: 1.0) // create our bee sprite node let bee = SKSpriteNode(imageNamed: "bee") // size our bee node bee.size = CGSize(width: 100, height: 100) // position our bee node bee.position = CGPoint(x: 250, y: 250) // attach our bee to the scene's node tree self.addChild(bee) }
Run the project and witness our glorious bee—great work!
